In the realm of React development, grasping the essentials of functional programming stands as a cornerstone for crafting applications that boast both simplicity and robustness. This guide illustrates the key principles of functional programming and showcases their implementation within React, empowering developers to write applications that are not only easier to debug but also to maintain. Whether you’re a React novice or looking to strengthen your understanding of this paradigm, this exploration will deepen your comprehension and skillset.
The Essence of Functional Programming
At its core, functional programming is a declarative paradigm centered on the assembly of pure functions to construct software. The notions of “composing” and “pure” functions are pivotal, serving as the foundation upon which functional programming rests.
The Purity of Functions
Consider a simple mathematical function where the output solely depends on the input. This relationship exemplifies a deterministic or pure function—unchanging and without side effects.
Pure Function Example:
function square(x) {
return x * x;
}
In this instance, every invocation with the same input will consistently yield the same output, illustrating the deterministic nature of pure functions.
Function Composition: The Symphony of Code
Function composition involves stitching together multiple functions to form intricate functionalities. This structural approach enhances code reusability and fosters maintainability, mirroring the harmonious principles observed in mathematical functions.
Functional Programming’s Influence in React
React’s ecosystem benefits immensely from functional programming principles, leveraging these concepts to enhance component reusability and application performance.
Embracing Pure Functions in React
A simple React component closely resembles a pure function, receiving props as inputs and rendering output based on these inputs. This purity allows for predictable components that render consistently across different environments.
The Power of Immutability and Pure Functions:
When dealing with React state, the paradigm advocates for treating data structures as immutable, ensuring that functions do not alter the original state but work on duplicates instead. This practice significantly reduces bugs and enhances performance.
Utilizing React.memo for Optimized Re-rendering
To mitigate unnecessary component re-renders, React introduces React.memo
, enabling developers to memoize components such that a re-render occurs only when props change. This technique epitomizes the judicious application of functional programming principles for performance gains.
The Art of State Handling in Functional Components
React encourages the use of functional programming concepts in state management, particularly when states depend on previous states. Employing callbacks within state updater functions ensures state changes remain pure and predictable.
const incrementCounter = (prevCount) => prevCount + 1;
By adhering to pure functions and immutability, developers circumvent common pitfalls, rendering applications more stable and efficient.
React Composition: Crafting Complex Interfaces
Composition in React mirrors the functional programming tenet of assembling larger, more complex components from smaller, reusable ones. This concept enables developers to construct flexible UIs by embedding components within components, much like stitching together functions for a bigger purpose.
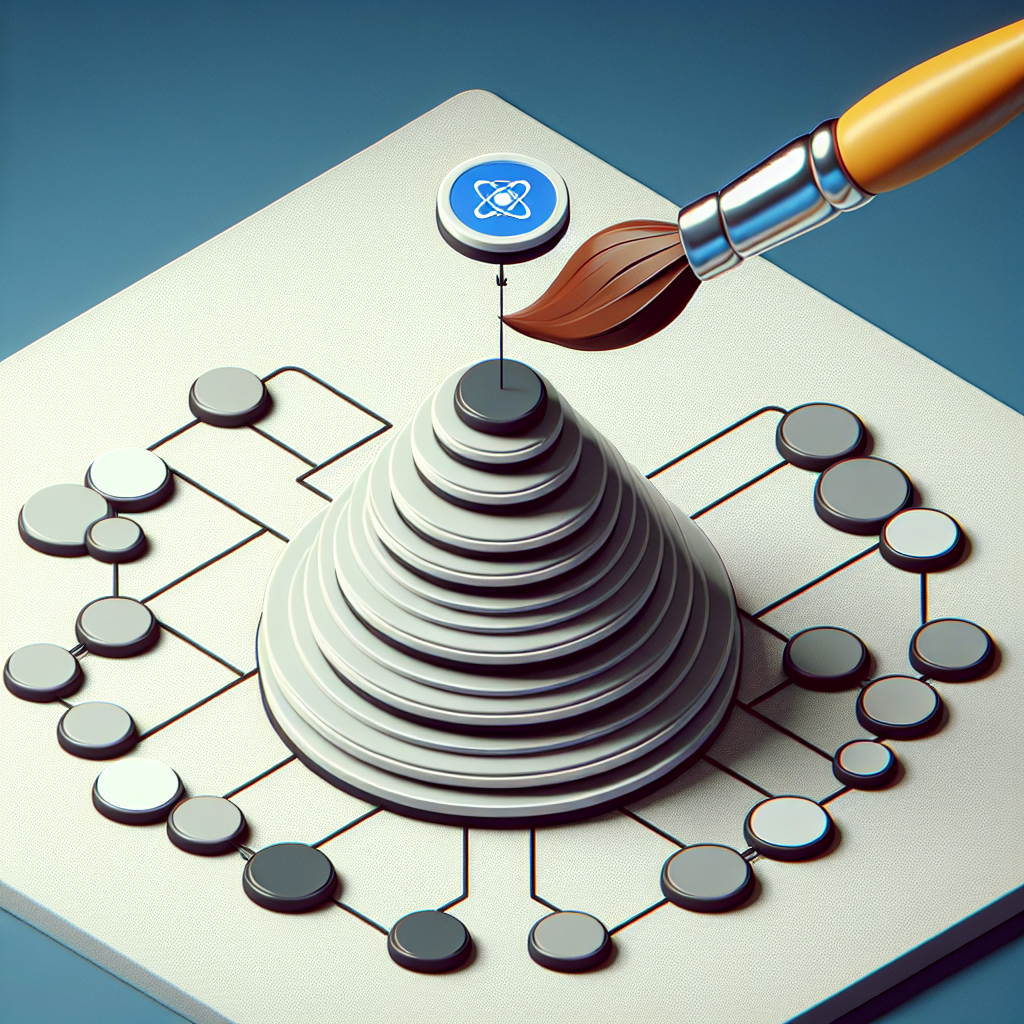
For example, a Hero
component might encapsulate varying content across different application sections, illustrating the dynamic and reusable nature of React components enabled by composition.
Wrapping Up: The Union of React and Functional Programming
Throughout this exploration, we’ve unveiled the symbiotic relationship between React and functional programming principles, emphasizing the importance of pure functions, immutability, and component composition. By adopting these concepts, React developers can craft applications that are not only scalable and maintainable but also poised for enhanced performance.
As you venture further into the world of React, let the principles of functional programming guide your journey, shaping the way you think about and structure your applications. Share your experiences and insights on this topic, and let’s continue to learn and grow together as a community.
Happy coding, and may your functions always be pure!