In the ever-evolving landscape of software development, understanding the underpinnings of data structures is not just an advantage—it’s a necessity. Dart and its popular UI toolkit Flutter have been gaining traction for developing high-fidelity, natively compiled applications for mobile, web, and desktop from a single codebase. This guide dives deep into the heart of Dart, unraveling the complexities of its data structures and demonstrating essential CRUD operations to elevate your programming competency.
List: Dart’s Flexible Arrays
Lists are among the most versatile and frequently used data structures in Dart. They represent an ordered collection of objects that can be accessed by their indices.
Creation and Modification
Creating a list in Dart can be as simple as using square brackets []
, which is the recommended way to create a growable list.
List<String> names = [];
For a fixed-size list populated with default values, Dart offers a filled constructor.
List<int> fixedList = List.filled(5, 0);
Operations
Retrieving an item from a list or modifying an element is straightforward; it involves using the item’s index.
String firstName = names[0]; // Retrieval
names[0] = "Alice"; // Modification
For dynamic modifications, Dart lists offer methods like add()
, remove()
, and setAll()
, enabling developers to manipulate lists with ease.
Map: Key-Value Pairs
Maps store objects as key-value pairs, allowing for efficient data retrieval and manipulation based on unique keys.
Initialization
Dart maps can be instantiated using curly braces {}
or the Map()
constructor, followed by adding entries either at initialization time or dynamically.
Map<String, int> ageMap = {'Alice': 30, 'Bob': 25};
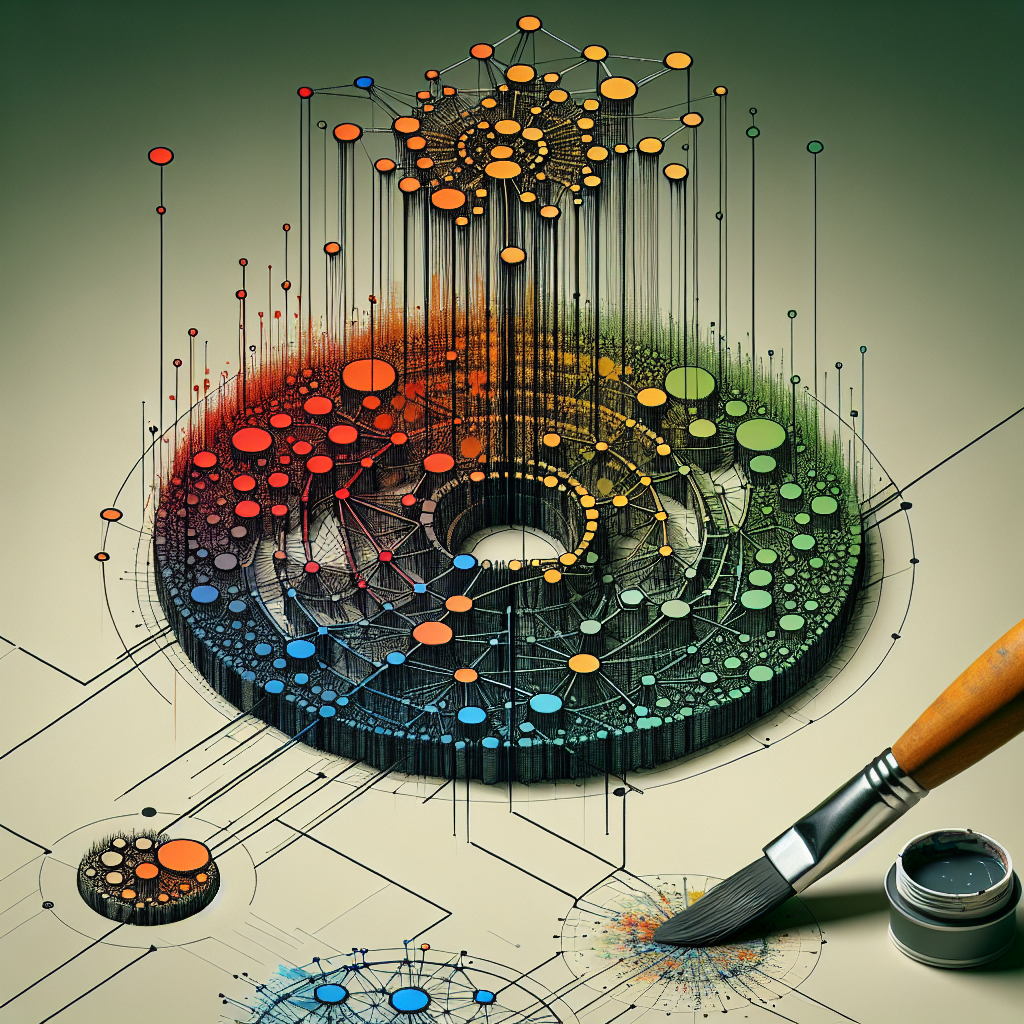
CRUD Operations
Adding or modifying entries in a map is intuitive, employing the key to access and alter its corresponding value.
ageMap['Charlie'] = 22; // Adding a new key-value pair
ageMap['Alice'] = 31; // Updating an existing value
Maps also provide efficient ways to remove entries and iterate through keys and values, ensuring robust data manipulation capabilities.
Set: Ensuring Uniqueness
Dart’s Set is a collection of unique elements, automatically eliminating duplicates and offering various implementations like HashSet
, LinkedHashSet
, and SplayTreeSet
.
Creation
Sets can be created using the Set()
constructor or set literals enclosed in curly braces {}
.
Set<int> numbers = {1, 2, 3, 4};
Operations
While directly updating elements in a set isn’t as straightforward as in lists or maps, Dart offers mechanisms to add, remove, and check the presence of elements efficiently.
numbers.add(5); // Adding a new element
numbers.remove(2); // Removing an element
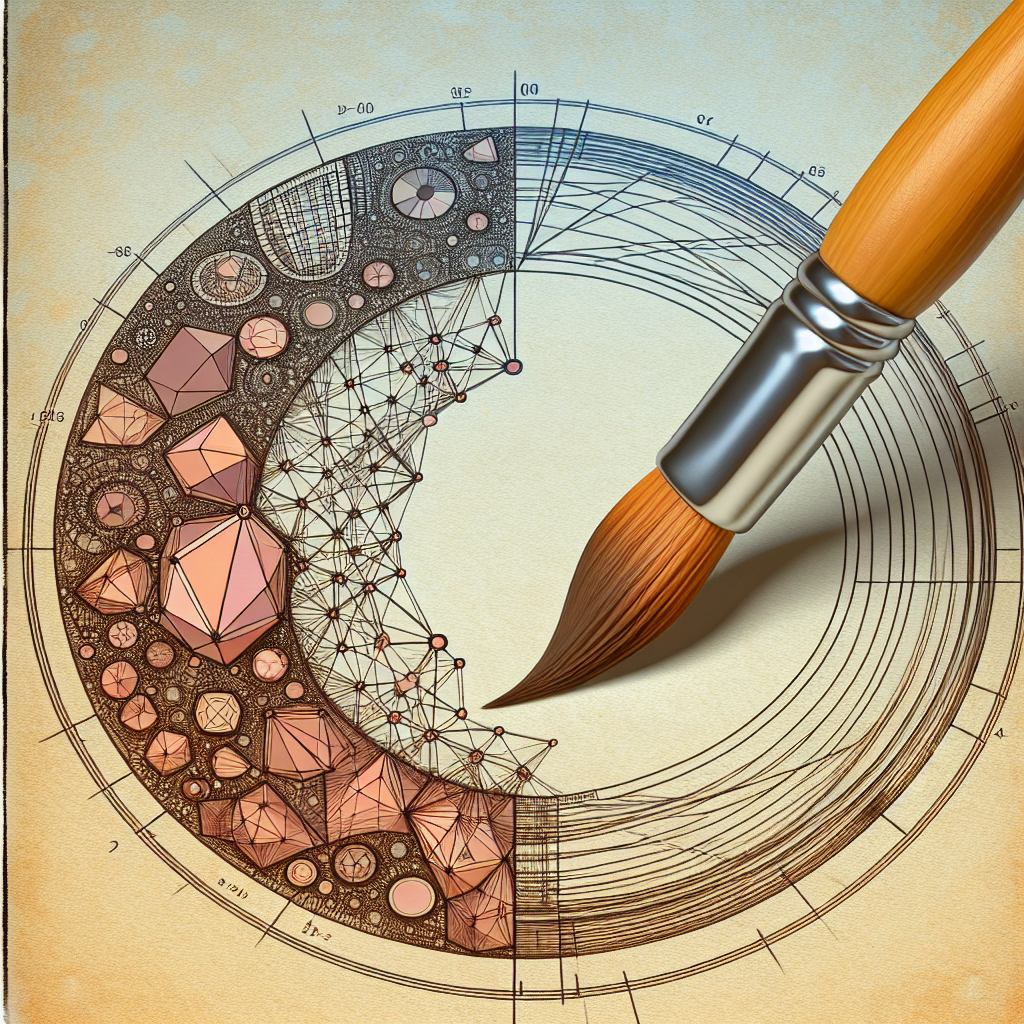
Choosing the Right Structure
When faced with a decision on which data structure to use, consider the operations your application will perform most frequently. Maps and sets are suited for unique value storage and efficient retrieval, while lists offer ordered storage with dynamic sizing.
Understanding the Operation Complexity can significantly influence performance, especially for applications dealing with large datasets.
Wrapping Up
Dart’s rich collection of data structures, combined with Flutter’s robust framework, provides a powerful toolkit for developing complex applications. By mastering these structures—lists, maps, and sets—you can optimize data handling and elevate your software development skills.
For further exploration and to sharpen your Dart and Flutter expertise, visit the official Dart documentation. Happy coding!