The realm of web development is continuously evolving, with new technologies emerging to solve existing challenges in more efficient ways. Among the groundbreaking advancements is the introduction of WebAssembly (Wasm), a binary instruction format that brings high-performance applications to the web. When combined with Rust, a language prized for its safety and speed, developers get a powerhouse duo that opens up new possibilities for web applications. This tutorial dives into the world of Wasm and Rust, guiding you through the steps to set up a Rust Wasm environment and illustrating how to leverage these technologies to boost your web applications.
Introduction to WebAssembly and Rust
WebAssembly (Wasm) is a supported binary format across most modern web browsers designed to execute code at near-native speed. It allows developers to compile languages like Rust, C, C++, and Go into a web-compatible format, enhancing performance without ditching JavaScript entirely. Instead, Wasm is best viewed as a complement to JavaScript, suitable for performing computationally intensive tasks more efficiently.
Rust, with its emphasis on safety and performance, has emerged as a preferred language for writing Wasm applications. Its compatibility with WebAssembly is not by chance but rather a conscious effort by the Rust team to support and advance Wasm as a first-class citizen in the web ecosystem.
To embark on this Wasm journey with Rust, ensure you have installed Rust and wasm-pack
on your machine. wasm-pack
is a toolchain designed to facilitate the assembly and packaging of Rust crates for WebAssembly, making it easier to integrate Rust code into your web projects.
Creating Your First Rust Wasm Project
To start, let’s create a Rust library ready to be compiled to WebAssembly. We’ll use wasm-pack
, which simplifies the process to just a few commands, mirroring the experience Rust developers are accustomed to with cargo
.
wasm-pack build --target web
is the magic command that targets our environment correctly, ensuring the output is ready to be used directly in web applications. This produces a set of files that act as the bridge between your Wasm binary and JavaScript.
Once your Rust code is compiled into Wasm, it’s straightforward to invoke it from JavaScript within the browser. However, to do so, we’ll serve our project files over a local web server, circumventing browser security restrictions against local file imports.
Bridging Rust and JavaScript with wasm-bindgen
Communication between Rust and JavaScript isn’t native; it requires a special crate called wasm-bindgen
. This tool facilitates interoperation, letting you call into JavaScript functions as if they were Rust functions and vice versa.
use wasm_bindgen::prelude::*;
[wasm_bindgen]
extern "C" {
pub fn alert(s: &str);
}
This snippet gives a taste of how wasm-bindgen
declares JavaScript functions for use in Rust, and it barely scratches the surface. For manipulating more complex types, such as enums and structs, or even working with JavaScript’s web APIs, wasm-bindgen
along with crates like web-sys
and js-sys
, offer a comprehensive solution.
Extending the Web with Rust
Beyond basic types and function calls, you can also hook into the vast array of web APIs available in browsers. Through the web-sys
crate, numerous APIs are at your fingertips, ranging from DOM manipulation to WebGL.
Below is a basic example of how one might begin integrating these web capabilities into a Rust Wasm project.
[dependencies]
web-sys = { version = "0.3.4", features = ["console"] }
This introduces web-sys with console feature enabled, allowing your Rust code to print messages directly to the browser’s console — a small but concrete step towards full-fledged web applications powered by Rust and Wasm.
Final Thoughts
The integration of WebAssembly and Rust in web development is not just about achieving performance gains; it’s about rethinking what’s possible on the web. This guide provided a gentle introduction, but the journey doesn’t end here. The ecosystem is vibrant and rapidly evolving, promising even more tools and libraries to make web development with Rust and Wasm a seamless experience.
While this post has outlined the basic steps towards a Rust-powered web application, I encourage you to dive deeper into the wasm-bindgen
and web-sys
documentation. There you’ll find the keys to unlock the full potential of high-performance web applications with Rust and WebAssembly.
Remember, this is just the beginning of your adventure with Rust and Wasm in the web development landscape. The possibilities are as vast as your imagination. Happy coding!
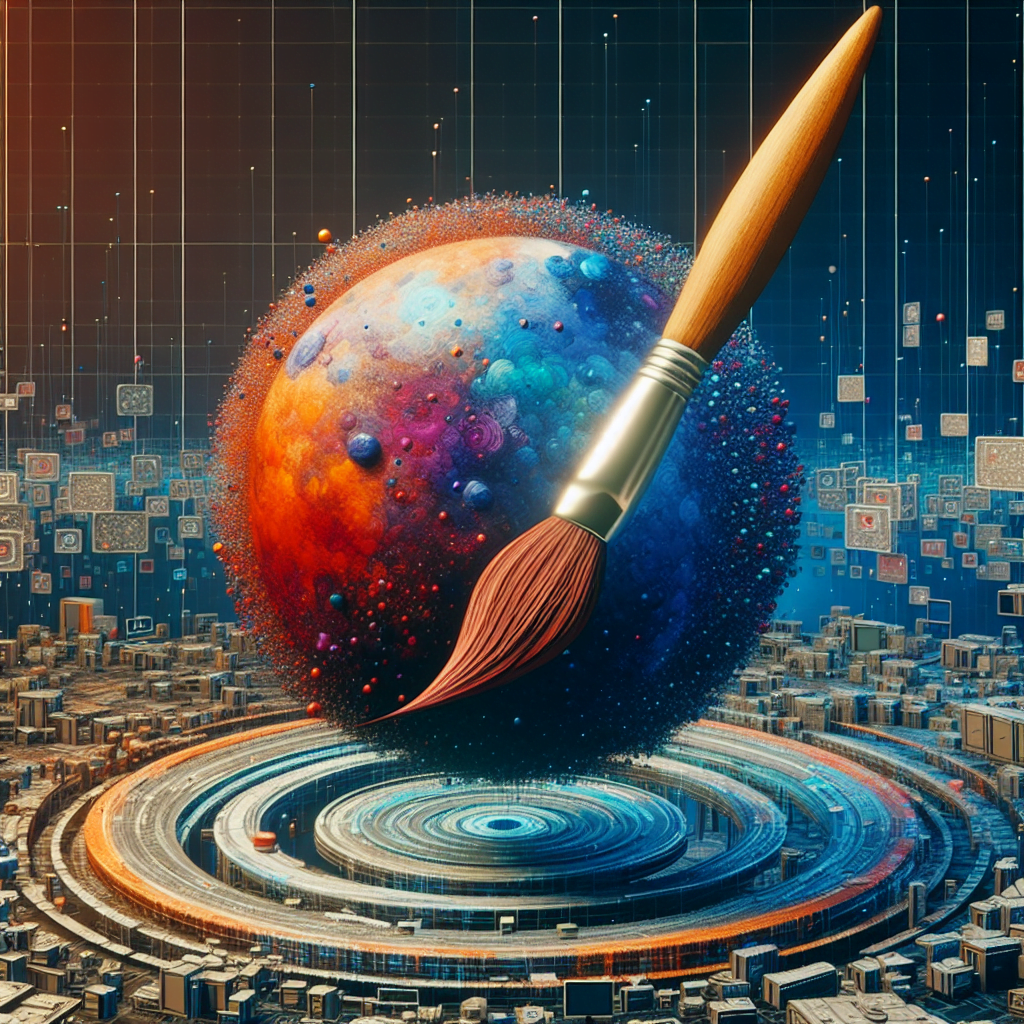